What is Superpowers?
Ever tried building a web app locally and hit CORS errors? Or needed to use API keys directly in your browser JavaScript without exposing them?
Superpowers is here to help. It's a Chrome extension that safely gives your local web pages—or any page you enable it on—extra abilities.
Fetch data from any domain without CORS headaches
Securely use API keys in your JavaScript
Call OpenAI's GPT models directly
Authenticate with Search Console, Analytics, and more
⚡️ Supercharge Your AI Prototyping
Building AI-powered apps typically requires complex backend setup, API key management, and dealing with CORS issues. Superpowers eliminates these hurdles, making it the perfect tool for rapidly prototyping applications that use AI APIs.
Run AI API calls directly from local HTML/JS without setting up a server
Store sensitive credentials like OPENAI_API_KEY
safely in the extension
Use Superpowers.OpenAI
for easy access to chat completions and image generation
Combine with the Superpowers Editor for extremely fast edit-test-debug cycles
From Idea to AI App in Minutes
Create functional AI prototypes in pure HTML/JS with this complete, runnable example:
<meta name=superpowers content=enabled>
<script src=https://superpowers.franzai.com/v1/ready.js></script>
<h1>GPT‑4o Chat</h1>
<div><input id=q placeholder=Ask><button id=b>➤</button></div>
<pre id=o>…</pre>
<script>
Superpowers.ready(async()=>{
if(!(await Superpowers.Env.getEnvVars()).OPENAI_API_KEY)
return o.textContent="Set OPENAI_API_KEY";
const ask=async()=>{
if(!q.value.trim())return;
o.textContent="⏳";
o.textContent=(await Superpowers.OpenAI.chatCompletion({
model:"gpt-4o",messages:[{role:"user",content:q.value}]
})).choices?.[0]?.message?.content||"🤖?";
};
b.onclick=ask;q.onkeyup=e=>e.key==="Enter"&&ask();
});
</script>
<style>
body{margin:0;display:grid;place-items:center;gap:.8em;height:100vh;
background:linear-gradient(135deg,#ffda6e 0%,#ff6cab 50%,#6be4ff 100%);
color:#fff;font:16px sans-serif}
input,button{padding:.4em .6em;border:0;border-radius:.4em;font:inherit}
button{background:#00c8ff;color:#fff}
pre{max-width:90vw;white-space:pre-wrap}
</style>
Try it for your next AI project! Combine Superpowers with the Superpowers Editor for the ultimate AI prototyping experience.
Quick Start
- Install the Extension: download it above and follow the installation steps.
- Enable in Your HTML: Add these two lines inside your
<head>
tag:<meta name="superpowers" content="enabled"> <script src="https://superpowers.franzai.com/v1/ready.js"></script>
- Use the API: wait for Superpowers to be ready:
Superpowers.ready(async () => { console.log("Superpowers Ready!"); try { const env = await Superpowers.Env.getEnvVars(); const response = await Superpowers.fetch('https://jsonplaceholder.typicode.com/todos/1'); const data = await response.json(); console.log({env, data}); } catch (err) { console.error("Superpowers API call failed:", err); } });
Installation Guide (Chrome)
- Download the Extension Files: grab the
.zip
using the button near the top. - Extract the ZIP File into a folder.
- Open Chrome Extensions: type
chrome://extensions
in the address bar. - Enable Developer Mode (top‑right).
- Load the Extension: click "Load unpacked" and pick the extracted folder.
- Done! The Superpowers icon now appears in your toolbar.
- Pin the Extension: click the puzzle piece icon in Chrome's toolbar, then pin Superpowers by clicking the pin icon next to it for easy access.
- Configure OpenAI API: click the Superpowers icon (circular shield) in your toolbar to open the side panel. Add your
OPENAI_API_KEY
in the Environment Variables section. You can get an API key from OpenAI if you don't have one.
Important: keep the extension folder intact—moving or deleting it disables the extension.
Extension Setup Preview
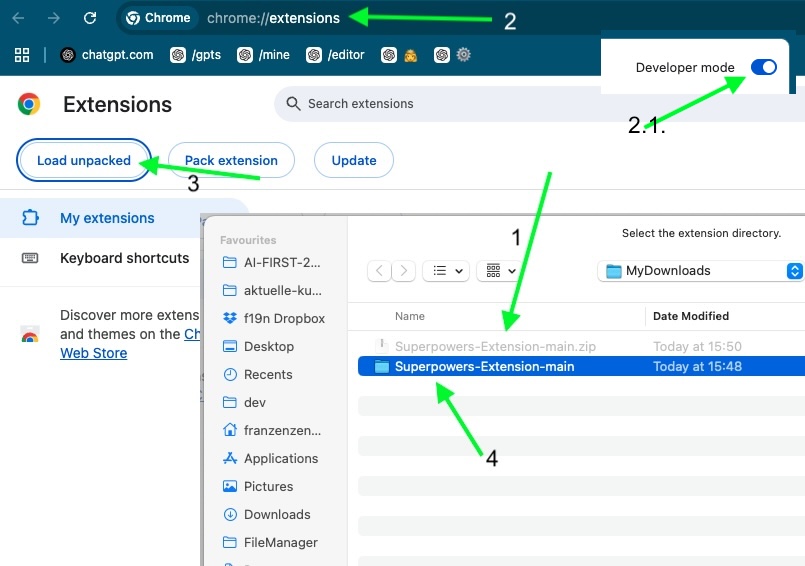
How to Use Superpowers
1. Enable in Your HTML
Add these two lines inside the <head>
:
<meta name="superpowers" content="enabled">
<script src="https://superpowers.franzai.com/v1/ready.js"></script>
2. Wait for Initialization
Superpowers loads asynchronously—always wrap your calls:
Superpowers.ready(() => {
// Safe to use Superpowers APIs
});
Superpowers.readyerror(err => console.error("Init failed:", err));
3. Access the Side Panel
- Environment Variables: add API keys & secrets
- Debug Output: view extension logs
- Credentials Manager: manage Google OAuth access
Key Features
Environment Variables
// inside Superpowers.ready()
const env = await Superpowers.Env.getEnvVars();
const apiKey = env.MY_API_KEY;
Cross‑Domain Fetch
const res = await Superpowers.fetch('https://api.github.com/users/octocat');
const data = await res.json();
AI Integration (OpenAI)
const completion = await Superpowers.OpenAI.chatCompletion({
model:"gpt-4o",
messages:[
{role:"system",content:"You are a helpful assistant."},
{role:"user",content:"Explain quantum physics simply."}
]
});
Superpowers Editor
Need a sandbox for quick experiments? Launch the web‑based editor:
Troubleshooting
- Extension not working? Check the
<meta>
tag is in<head>
. - API calls failing? Ensure you wrapped them in
Superpowers.ready()
. - AI calls failing? Verify your API key in the side panel.
- Need help? See the GitHub repo.